Variables & Datatypes
JavaScript variables are simply data containers. They come in many forms ...
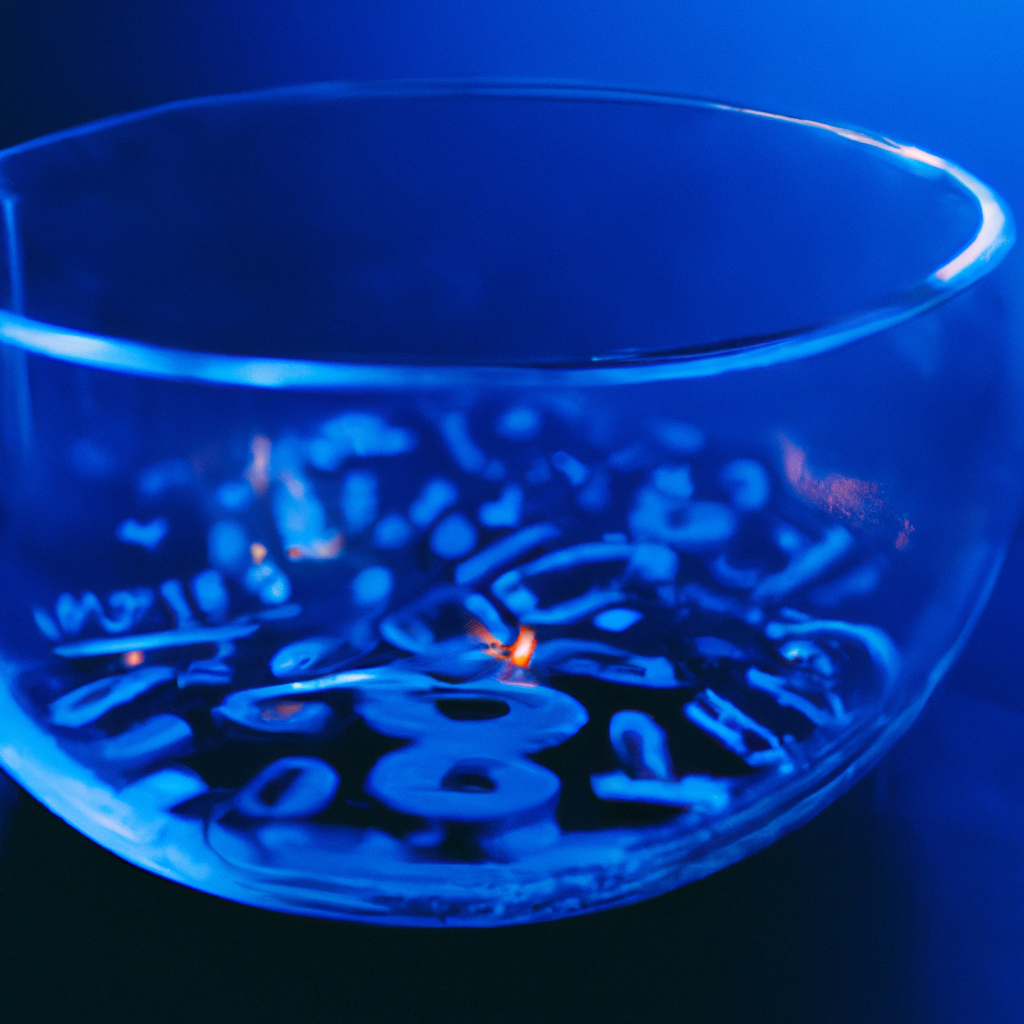
JavaScript Beginner Series
- JavaScript Introduction
- Variables and Datatypes
In this article, we'll jump into one of the building blocks of JavaScript, variables.
What are variables? Computer memory is one big sea of bits: zeros, and ones. A variable is a name you give to reference a particular location in this vast sea. A location where you intend to put a piece of data that you can later reference. Think of a variable as a container in the computer memory.
Data types
What can you put in these containers? Any piece of data that this a valid JavaScript data type. JavaScript has five basic data types: String, Number, Boolean, Undefined, Null, BigInt, and Symbol. There are also other compound data types such as objects and arrays.
So how do you actually structure a variable? To begin, you need to declare it and then initialize it with a value/ or assign a value to it. For example,
- Declaration - Where you give your variable name. You use the keywords
var, let
orconst
to do this example:
var myVar;
let myVar;
2. Assignment - where you assign a value to the variable. You use the assignment operator =
to do this.
myVar = 12;
myVar = "Hello, world";
The two steps can be combined into one:
let myVar = 12;
In the case of const, its value must be assigned during declaration because it cannot change.
const myVar = "Hello, world";
var firstName = "John";
or
let firstName = "James";
or
const firstName = "Myers";
There are several naming conventions used when giving a variable its name:
camelCase
where you start with a lower case and the second word begins in uppercase, PascalCase
and snake_case
. We will mostly use camelCase in these articles, but feel free to look up the others and use what appeals to you.
There are a few things to keep in mind about variables.
- The first is every variable has a name, called its identifier. This is just a series of characters that link to a variable.
- In JavaScript, identifiers are case-sensitive. This means that
container
is different fromContainer
. - Third, identifiers can only contain letters, numbers, the underscore
_
, or the dollar sign$
. An identifier cannot begin with a number. It can only begin with either a letter, the underscore, or the dollar sign. - An identifier cannot be any of the JavaScript-reserved words.
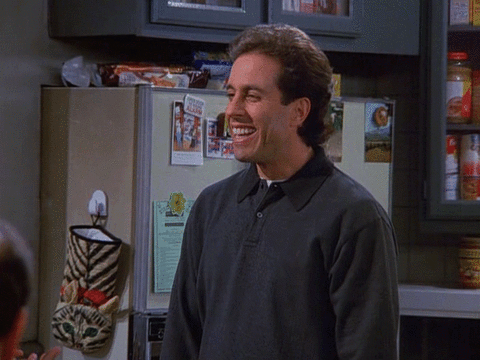
Now, these days, from 2015 onwards, let and const are commonly used since they were introduced in the new version of JavaScript, ES6. Before that, var was used but because of some of its nuances, the newer keywords are preferred. Let's take a closer look at these keywords.
- const, it is used to declare a constant, i.e. a fixed and non-changing value. So once you define it, it cannot change.
- let, it is used to declare a variable that has the potential to change in the future, (you mostly use this). The same definition can be applied to var.
//For example:
let lastName = "Doe";
//later on we can change the value of last name,
lastName = "Brown";
//Note we don't need to redeclare the variable with the let keyword again.
Why don't we use the var keyword as much these days? It has to do with something known as scoping. In a nutshell, we have a global scope and a local scope, we'll dive deeper into this later on. But for clarity's sake, a variable declared with var in the local scope can be accessed on the global scope, and sometimes you don't want this behavior.
It's also important to keep in mind that:-
- You can declare multiple variables in one statement, for example:
- A declared variable without a value assigned to it will result in the value
undefined
, Â for example: - If you redeclare a variable with
var
, the initial value will be upheld but if you do so withlet
andconst
you will get a Syntax Error: Identifier 'the identifier you input' has already been declared. Example:
let firstName = "John", lastName = "Doe", age = 26;
//separate the variables with a comma.
let houseNumber;
//output will be undefined.
var houseName = "BlockA";
var houseName = "BlockB";
//output will be BlockB
let brandName = "Safaricom";
let brandName = "OtherBrand";
//A syntax error will be thrown.
Now earlier I touched on data types briefly, so let me give you the complete rundown on them. There are 7 primitive data types in JavaScript. Primitive meaning, data that isn't an object and doesn't have any methods or properties. These are String, Number, Boolean, Null, Undefined  BigInt, and Symbol. In this article, we won't deep dive into Symbol but you can read more about here, and BigInt
but you can learn more about it in this article. So let's break the others down.
- A string, Â it's a collection of characters enclosed by delimiters i.e. single quotes or double quotes. Such a collection of characters is known as a string datatype.
- A number, this is simply a numerical value. It's a foundational part of JavaScript because it represents both integers and decimal point numbers.
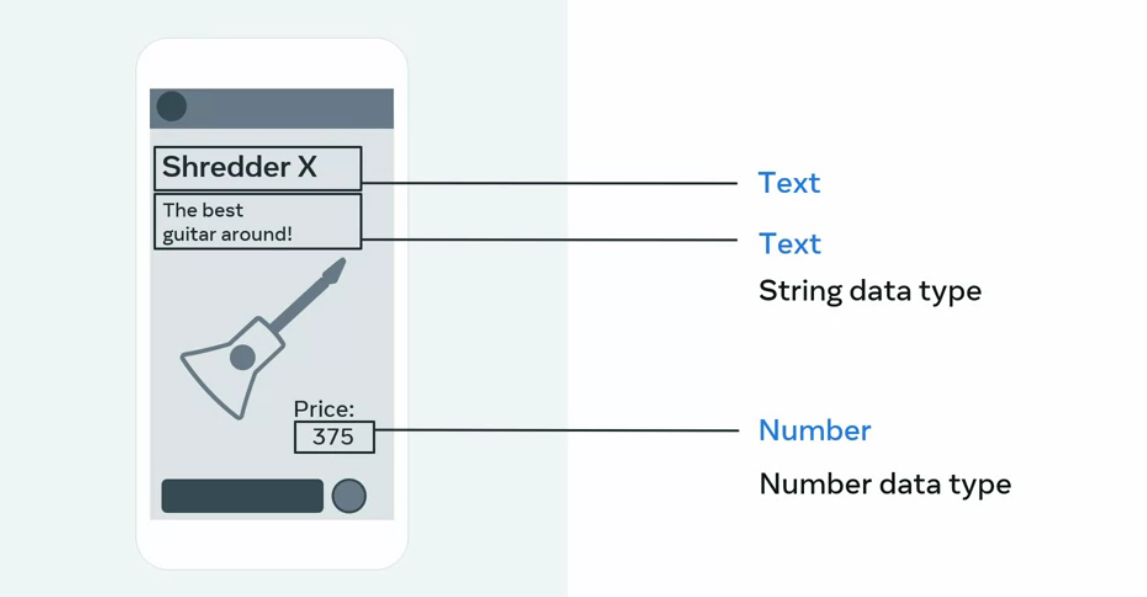
3. Boolean, is a data type that has only one of two possible values, true
or false
. It's useful for making decisions, for example here you can pair it with a strict equality operator(covered in the next article):
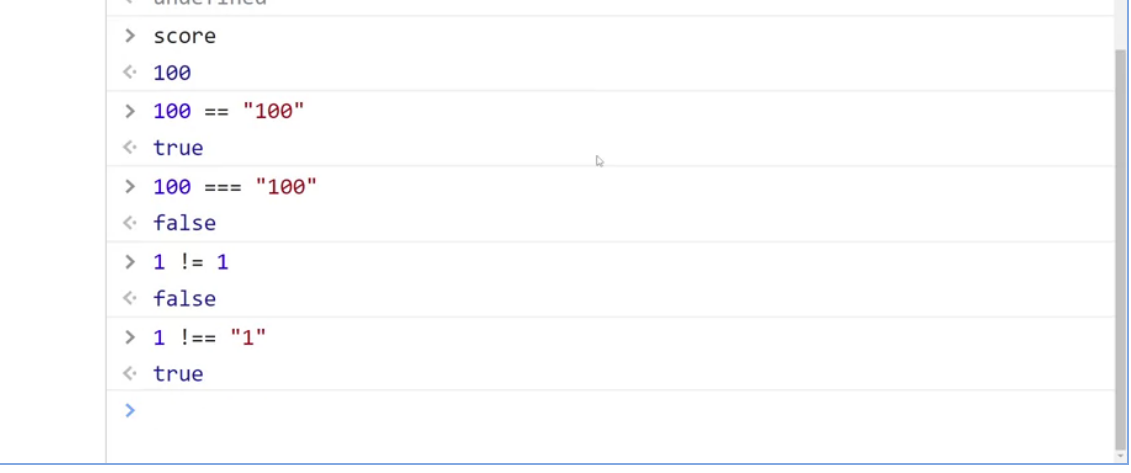
4. Null, represents the absence of value. Note that, a variable is automatically given the value null
if it is created without a value.
5. Undefined, can only hold the value undefined
and refers to a variable that hasn’t been assigned a value.
But you may be wondering Null and Undefined seem similar, well, while Undefined denotes the value is not specified by the compiler, Null denotes the value is intentionally absent.
If you find this article enjoyable and helpful, you subscribe to my newsletter, Just in Time, it's free and I can send you more nuggets of wisdom like this. It'll help support my work and continue to publish well-curated posts. Thank you!