Javascript operators - Part I
JavaScript operators, simply put, are used to manipulate variables and their values, and together they form an expression.
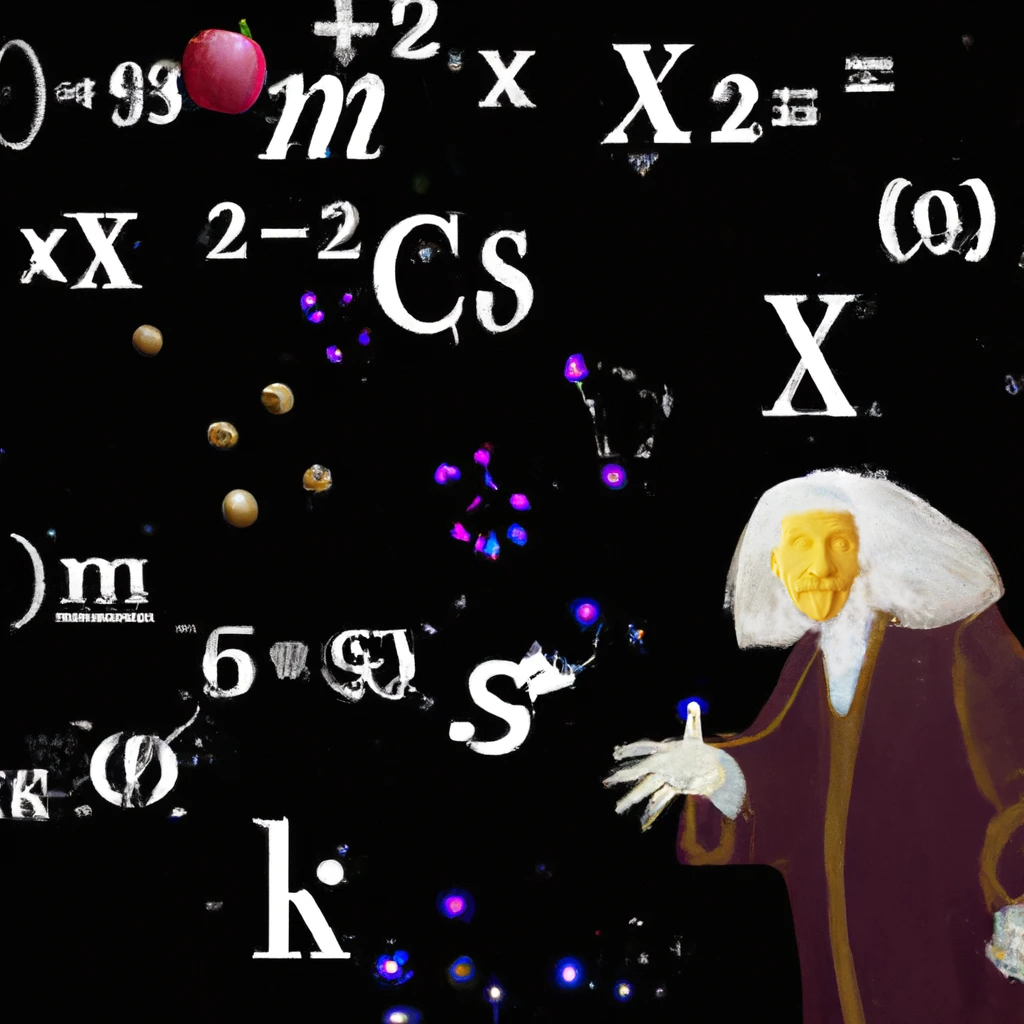
What are JavaScript operators?
Simply put, they are used to manipulate variables and their values and together they form an expression. In general, an expression is a combination of values and functions that may be evaluated to create a new value. For example:
let a = 2;
let y = 5;
a = a + y;
console.log(a); // output is 7.
/* In this example, the = sign is called an assignment operator.
It assigns the value on the right hand side to the variable on the left hand side. */
There are three main types of operators in JS, arithmetic, comparison, and logical operators. Let's go through them.
Arithmetic operators
Even if you're new to programming, I bet you're familiar with these operators. They include addition (+), subtraction (-), division (*), multiplication ( / ), and the modulus operator (%), also known as the remainder operator.
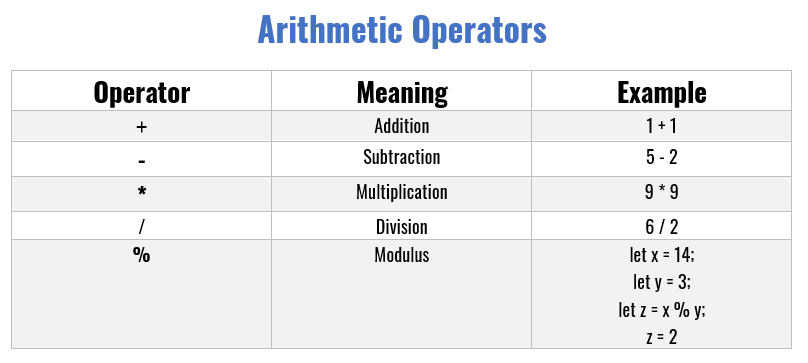
These are all self-explanatory but one needs further explanation. Let's take a closer look at the modulus operator. What it does is just return the remainder of a division operation. In the example above, the value of y goes into x 4 times, which evaluates to 12, and the remainder is 2.
It's important to note that the addition operator can also be used on strings although it'll have unusual behavior. When used on/with strings it performs string concatenation. Meaning it stitches the strings into a single string. For example:
let greet = "Hello";
let fName = "Mike";
let newGreeting = greet + " " + fName;
console.log(newGreeting);
// output is Hello Mike
It can also be used to concatenate strings with numbers that output a string. For example:
let num = 5;
let sNum = "5";
let newNum = num + sNum;
console.log(newNum);
// output is "55";
// If we then concatenate that output with a number it results in this
let newNum = num + sNum;
let cNum = newNum + 10;
console.log(cNum);
// output is "5510" which is a string.
// We can confirm this is a string by using the typeOf operator on the output.
Comparison Operators
A comparison expression returns a boolean value indicating either true or false.
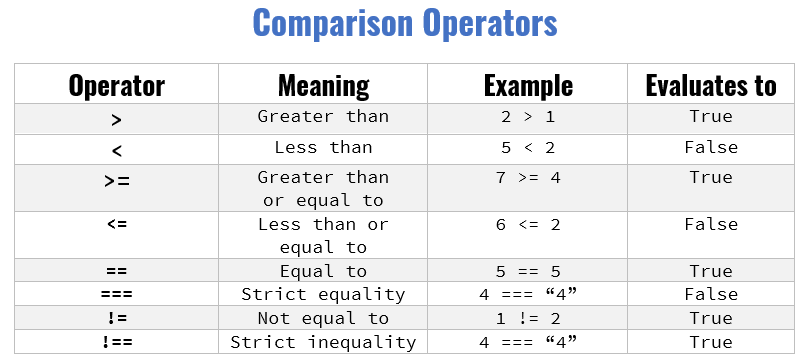
Some of these are straightforward but a few warrant more explanation.
- Let's look at the equality operator. It checks if two values are equal. A common mistake is using the ( = ) assignment operator to evaluate equality.
- Next, the strict equality operator ( === ) compares both the values and the data types. Thus when comparing
5 === 5
, this returns true. The values on each side of the strict equality operator have the same value and the same type. However, comparing5 === "5"
now returns false, because although the values are equal, the data types are different. - The other one is the inequality operator ( != ). It checks if two values are not the same, but it does not check against the difference in types. For example,
5 != "5"
returns false, because it's false to claim that the number 5 is not equal to the number 5, even though this other number is of the string data type. The table above1 != 2
evaluates to true because it's true that the number 1 isn't equal to the number 2.
Thus the strict inequality operator ( !== ) compares both the value and the data type. So for the strict inequality operator to return false, the compared values have to have the same value and the same data type. For example, 4
!==
4
returns false because it is false to say that the number 4 is not of the same value and data type as another number 4.
However, comparing the number 4 and the string 4, 4 !== "4
", using the strict inequality operator, returns true.
Logical Operators
In simple terms, logical operators perform logic. When used together with control statements and comparison operators they implement a more complicated logic.
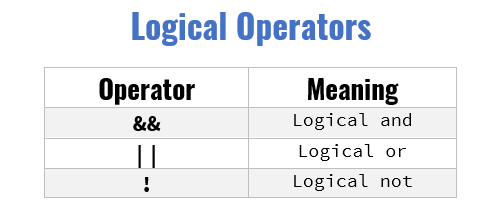
Let's take a closer look at them.
- The logical AND operator ( && ) denoted with a double ampersand sign is used to confirm if multiple comparisons will return true. It returns true if both comparisons are true and it returns false if one of the values is false or if both are false. For example:
let speed = 10;
console.log(speed > 8 && speed < 15);
// returns true
console.log(speed > 10 && speed < 5);
// returns false because both statements are false
console.log(speed > 10 && speed < 15);
// returns false because one statement is false
- The logical OR operator ( || ) denoted by a double pipe sign is used to check if at least one of the given comparisons evaluates to true. If both comparisons are true, or one is true it will return true. But if both are false the output will be false. Using the snippet above here's an example:
let speed = 10;
console.log(speed > 8 || speed < 15);
// returns true
console.log(speed > 10 || speed < 5);
// returns false because both statements are false
console.log(speed > 10 || speed < 15);
// returns true because one statement is true i.e. (false || true)
- Now, exclamation mark what is it? In JS it's the logical NOT operator. You can think of it as a switch that flips the evaluated boolean value to the opposite i.e. true to false and vice versa. For example:
let babyHungry = true;
console.log('Feeding the baby');
console.log("Baby is hungry: ", !babyHungry);
console.log(babyHungry);
// Try it out and see the output.
Now that that is out of the way, let me give you an example of how you would use the logical operators in real projects using if statements, which we will cover in a later article.
Let's write a small program that turns the lights on if the speed is above 10 and the passengers are less than 6.
let speed = 12;
let passengerNum = 8;
let lightsOn = false;
if (speed >= 10 && passengerNum > 6 ) {
console.log(!lightsOn);
} else {
console.log("No need to turn lights on.");
}
The above snippet returns true. Why? Because we are telling the console if speed is above or equal to 10 AND the number of passengers is above 6, return whatever the lightsOn
is NOT. Since the above conditions are met i.e both expressions are true ( true && true ), the logical NOT operator logs the opposite value of the lightsOn
boolean value i.e. true. Try changing the values of speed and passengerNum on the variables and check the result.
These are some of the fundamental building blocks of JavaScript and understanding them will be critical in your journey.
If you find this article enjoyable and helpful, you subscribe to my newsletter, Just in Time, it's free and I can send you more nuggets of wisdom like this. It'll help support my work and continue to publish well-curated posts. Thank you!